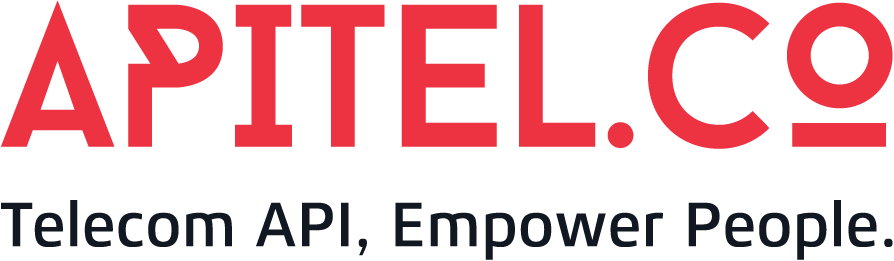
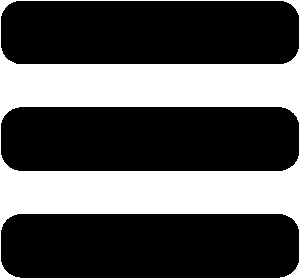
ส่ง SMS
คุณสามารถส่งข้อความ โดยกำหนดหมายเลขผู้รับและชื่อผู้ส่งได้ ในการส่ง SMS ให้ส่งคำขอ POSTไปที่ /sms endpoint
การส่งคำขอ
URL | https://api.apitel.co/sms |
Method | POST |
Content type | application/x-www-form-urlencoded หรือ application/json |
พารามิเตอร์
ข้อความที่ต้องการส่งไปยังหมายเลขผู้รับ ข้อความควรเข้ารหัสเป็น UTF-8 เพื่อรองรับทุกภาษา
ข้อความที่ประกอบด้วยชุดตัวอักษร (Character Set) ในรูปแบบของ ISO-Latin-1 เท่านั้นสามารถมีความยาวได้สูงสุด 160 ตัวอักษร หรือสูงสุด 153 ตัวอักษร สำหรับข้อความที่ถูกตัดเป็นหลายๆข้อความ
ข้อความที่ประกอบด้วยชุดตัวอักษร (Character Set) ในรูปแบบอื่น ๆ สามารถมีความยาวได้สูงสุด 70 ตัวอักษร หรือสูงสุด 67 ตัวอักษร สำหรับข้อความที่ถูกตัดเป็นหลายๆข้อความ
ข้อความที่ถูกตัดเป็นหลายๆข้อความจะถูกรวมและแสดงเป็นข้อความเดียวบนอุปกรณ์ผู้รับ
ตัวอย่าง
curl -i -X POST "https://api.apitel.co/sms" \
-H "Content-Type:application/json" \
-d \
'{
"to": "+661234567890",
"from": "ATSMS",
"text": "A text message",
"apiKey": "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
"apiSecret": "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
}'
const fetch = require('node-fetch');
const body = {
to: '+661234567890',
from: 'ATSMS',
text: 'A text message',
apiKey: 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx',
apiSecret: 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
};
fetch('https://api.apitel.co/sms', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(body)
})
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error(err));
$data = array(
"to" => "+661234567890",
"from" => "ATSMS",
"text" => "A text message",
"apiKey" => "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
"apiSecret" => "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
);
$data_string = json_encode($data);
$ch = curl_init('https://api.apitel.co/sms');
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "POST");
curl_setopt($ch, CURLOPT_POSTFIELDS, $data_string);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json',
'Content-Length: ' . strlen($data_string))
);
$result = curl_exec($ch);
$http_code = curl_getinfo( $ch, CURLINFO_HTTP_CODE );
if ( $http_code == "200" ){
echo 'Successful! Server responded with:'.$result;
}else{
echo 'Failed! Server responded with:'.$result;
}
curl_close( $ch );
require 'net/http'
require 'uri'
require 'json'
uri = URI.parse("https://api.apitel.co/sms")
header = {'Content-Type': 'application/json'}
body = {
to: "+661234567890",
from: "ATSMS",
text: "A text message",
apiKey: "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
apiSecret: "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
}
http = Net::HTTP.new(uri.host, uri.port)
request = Net::HTTP::Post.new(uri.request_uri, header)
request.body = body.to_json
response = http.request(request)
if response.is_a?(Net::HTTPSuccess)
puts "Successful! Server responded with: #{response.body }"
else
puts "Failed! Server responded with: #{response.body }"
end
import requests
body = {
'to': "+661234567890",
'from': "ATSMS",
'text': "A text message",
'apiKey': "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
'apiSecret': "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
}
r = requests.post('https://api.apitel.co/sms', json=body)
if r.status_code >= 200 and r.status_code < 300:
print('Successful! Server responded with:', r.json())
else:
print('Failed! Server responded with:', r.json())
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.io.IOException;
// This example requires Apache HTTP Components dependency,
// you can access the full documentation for more examples from link below.
// http://hc.apache.org/httpcomponents-client-ga
// http://hc.apache.org/httpcomponents-client-ga/examples.html
public class SendSmsMain {
public static void main(String[] args) throws IOException {
SendSms();
}
public static void SendSms() throws IOException {
HttpClient httpclient = HttpClients.createDefault();
HttpPost httppost = new HttpPost("https://api.apitel.co/sms");
String body = "{\"to\":\"+661234567890\"" +
", \"from\":\"ATSMS\"" +
",\"text\":\"A text message\"" +
",\"apiKey\":\"xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx\"" +
",\"apiSecret\":\"xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx\"}";
httppost.setEntity(new StringEntity(body, "UTF-8"));
httppost.addHeader("Content-Type", "application/json");
HttpResponse response = httpclient.execute(httppost);
HttpEntity entity = response.getEntity();
String responseBody = entity != null ? EntityUtils.toString(entity) : null;
int status = response.getStatusLine().getStatusCode();
if (status >= 200 && status < 300) {
System.out.println("Successful! Server responded with:" + responseBody);
} else {
System.out.println("Failed! Server responded with:" + responseBody);
}
}
}
using System;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
namespace apitel_test
{
class Program
{
static void Main(string[] args)
{
SendSmsAsync().Wait();
}
private static async Task SendSmsAsync()
{
using (HttpClient client = new HttpClient())
{
var url = "https://api.apitel.co/sms";
var body = "{\"to\":\"+661234567890\"" +
", \"from\":\"ATSMS\"" +
",\"text\":\"A text message\"" +
",\"apiKey\":\"xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx\"" +
",\"apiSecret\":\"xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx\"}";
var payload = new StringContent(body, Encoding.UTF8, "application/json");
var response = await client.PostAsync(url, payload);
using (HttpContent content = response.Content)
{
string result = await content.ReadAsStringAsync();
if (response.StatusCode == System.Net.HttpStatusCode.OK)
{
Console.WriteLine("Successful! Server responded with:" + result);
}
else
{
Console.WriteLine("Failed! Server responded with:" + result);
}
}
}
}
}
}
การตอบรับ
การตอบรับจะถูกจัดรูปแบบเป็น JSON เมื่อมีการส่งคำขอสำเร็จ จะมีตอบกลับเป็น HTTP status code: 200 OK แต่ถ้าหากมีข้อผิดพลาดเกิดขึ้นก็จะมีตอบกลับเป็น HTTP status code: 400 Bad Request
Keys
ตัวอย่าง
การตอบรับเมื่อส่งข้อความสำเร็จ
{
"id": 13,
"from": "ATSMS",
"to": "+661234567890",
"text": "Good evening.",
"status": "ACCEPTED"
}
การตอบรับเมื่อส่งข้อความยาวสำเร็จ โดยข้อความยาวจะถูกตัดเป็น 2 ข้อความ
{
"id": 91,
"from": "ATSMS",
"to": "+661234567890",
"text": "Good evening. There is a lot to say in this message. I suspect it may be too long to fit in a single sms. Unfortunately, they're limited in length to only 140 bytes.",
"status": "ACCEPTED",
"segments":[
{
"id": 91,
"text": "Good evening. There is a lot to say in this message. I suspect it may be too long to fit in a single sms. Unfortunately, they're limited in length to onl"
},
{
"id": 92,
"text": "y 140 bytes."
}
]
}
การตอบรับเมื่อส่งข้อความไม่สำเร็จ สิ่งนี้อาจเกิดขึ้นในกรณีระบุ content type ที่ไม่ถูกต้อง หรือ json syntax ไม่ถูกต้อง
{
"errors": {
"request": [
"Unable to parse request"
]
}
}
การตอบรับเมื่อส่งข้อความไม่สำเร็จ สิ่งนี้อาจเกิดขึ้นในกรณีไม่ระบุพารามิเตอร์ที่จำเป็น
{
"errors": {
"from": [
"can't be empty"
]
}
}
สถานะ SMS
ในการรับรายละเอียดสถานะของข้อความที่ส่ง คุณจะต้องมีระบบที่รองรับ webhook จากนั้นคุณจะต้องกำหนดค่าที่บัญชีของคุณเพื่อส่งการแจ้งเตือนสถานะไปยัง URL ที่กำหนดไว้ ตัวอย่างเช่น http://yourdomain.com/sms-status
ตัวอย่าง
const express = require('express')
const app = express()
app.route('/sms-status').get(function(request, response) {
handleStatus(request.query, response)
});
function handleStatus(query, response) {
console.log('message id', query.id);
console.log('message status', query.status);
response.sendStatus(200)
}
app.listen(8080);
$id = $_GET['id'];
$status = $_GET['status'];
$text = "message id: " . $id . " message status: " . $status;
// change this to a file which the php process can write to
$file = "/path/to/status_log.txt";
file_put_contents($file, $text, FILE_APPEND | LOCK_EX);
http_response_code(200);
# http://sinatrarb.com/
require 'sinatra'
get '/' do
id = params["id"]
status = params["status"]
puts "message id: #{id}"
puts "message status: #{status}"
[200, ""]
end
from flask import Flask, request, make_response
app = Flask(__name__)
def sms_status():
id = request.args.get('id')
status = request.args.get('status')
print('message id:', id)
print('message status:', status)
return make_response("", 200)
// http://sparkjava.com/
import static spark.Spark.*;
public class SMSStatus {
public static void main(String[] args) {
port(8080);
get("/", (request, response) -> {
String id = request.queryParams("id");
String status = request.queryParams("status");
System.out.println("message id: "+ id);
System.out.println("message status: "+ status);
response.status(200);
return "";
});
}
}
// http://nancyfx.org/
namespace NancyTemplate
{
using Nancy;
using System;
public class HomeModule : NancyModule
{
public HomeModule()
{
Get("/", parameters => {
var id = Request.Query.id;
var status = Request.Query.status;
Console.WriteLine("message id: "+ id);
Console.WriteLine("message status: "+ status);
return HttpStatusCode.OK;
});
}
}
}
สถานะการส่งคำขอ webhook จะประกอบไปด้วย 'id' และสถานะของข้อความ 'status' ตัวอย่างเช่น http://yourdomain.com/sms-status?id=1&status=DELIVERED
สถานะพารามิเตอร์
ในกรณีที่ข้อความไม่สามารถส่งถึงผู้รับจะแสดงบนหน้่า dashboard ของคุณ คำอธิบายแต่ละสาเหตุเป็นดังข้างล่างนี้
Undelivered status
ในกรณีของ 'Expired' การส่งข้อความอีกครั้งอาจประสบความสำเร็จ ในกรณีอื่นๆการส่งข้อความอีกครั้งมีความเป็นไปได้สูงที่จะไม่ประสบความสำเร็จ
Send SMS Batch
คุณสามารถส่งชุดข้อความไปยังหลายหมายเลขได้ในการส่งครั้งเดียว ให้ส่ง POST request ไปที่ /sms-batch endpoint
Request
URL | https://api.apitel.co/sms-batch |
Method | POST |
Content type | application/json |
Parameters
อาร์เรย์ของชุดข้อมูล (Message object) สามารถส่งได้สูงสุด 10000 ข้อความต่อ 1 ชุด
ชื่อของชุดข้อความของคุณ มีความยาวได้สูงสุด 20 ตัวอักษร
Message object
ข้อความที่ต้องการส่งไปยังหมายเลขผู้รับ ข้อความควรเข้ารหัสเป็น UTF-8 เพื่อรองรับทุกภาษา
ข้อความที่ประกอบด้วยชุดตัวอักษร (Character Set) ในรูปแบบของ ISO-Latin-1 เท่านั้นสามารถมีความยาวได้สูงสุด 160 ตัวอักษร หรือสูงสุด 153 ตัวอักษร สำหรับข้อความที่ถูกตัดเป็นหลายๆข้อความ
ข้อความที่ประกอบด้วยชุดตัวอักษร (Character Set) ในรูปแบบอื่นๆ สามารถมีความยาวได้สูงสุด 70 ตัวอักษร หรือสูงสุด 67 ตัวอักษร สำหรับข้อความที่ถูกตัดเป็นหลายๆข้อความ
ข้อความที่ถูกตัดเป็นหลายๆข้อความจะถูกรวมและแสดงเป็นข้อความเดียวบนอุปกรณ์ผู้รับ
Examples
curl -i -X POST "https://api.apitel.co/sms-batch" \
-H "Content-Type:application/json" \
-d \
'{
"from":"ATSMS",
"apiKey":"xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
"apiSecret":"xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
"messages":[
{"to":"+661234567890","text":"A first text message"},
{"to":"+661234567891","text":"A second text message"}
]
}'
const fetch = require('node-fetch');
const body = {
from: 'ATSMS',
apiKey: 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx',
apiSecret: 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx',
messages: [{
to: '+661234567890',
text: 'A first text message'
},
{
to: '+661234567891',
text: 'A second text message'
}
]
}
fetch('https://api.apitel.co/sms-batch', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(body)
})
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error(err));
$data = array(
"from" => "ATSMS",
"apiKey" => "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
"apiSecret" => "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
"message" => array(
array("to" => "+661234567890", "text" => "A first text message"),
array("to" => "+661234567891", "text" => "A second text message"),
)
);
$data_string = json_encode($data);
$ch = curl_init('https://api.apitel.co/sms-batch');
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "POST");
curl_setopt($ch, CURLOPT_POSTFIELDS, $data_string);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json',
'Content-Length: ' . strlen($data_string))
);
$result = curl_exec($ch);
$http_code = curl_getinfo( $ch, CURLINFO_HTTP_CODE );
if ( $http_code == "200" ){
echo 'Successful! Server responded with:'.$result;
}else{
echo 'Failed! Server responded with:'.$result;
}
curl_close( $ch );
require 'net/http'
require 'uri'
require 'json'
uri = URI.parse("https://api.apitel.co/sms-batch")
header = {'Content-Type': 'application/json'}
body = {
from: 'ATSMS',
apiKey: 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx',
apiSecret: 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx',
messages: [{
to: '+661234567890',
text: 'A first text message'
},
{
to: '+661234567891',
text: 'A second text message'
}
]
}
http = Net::HTTP.new(uri.host, uri.port)
request = Net::HTTP::Post.new(uri.request_uri, header)
request.body = body.to_json
response = http.request(request)
if response.is_a?(Net::HTTPSuccess)
puts "Successful! Server responded with: #{response.body }"
else
puts "Failed! Server responded with: #{response.body }"
end
import requests
body = {
'from': "ATSMS",
'apiKey': "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
'apiSecret': "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
'messages': [
{'to': "+661234567890",'text': "A first text message"},
{'to': "+661234567891",'text': "A second text message"}
]
}
r = requests.post('https://api.apitel.co/sms-batch', json=body)
if r.status_code >= 200 and r.status_code < 300:
print('Successful! Server responded with:', r.json())
else:
print('Failed! Server responded with:', r.json())
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.io.IOException;
// This example requires Apache HTTP Components dependency,
// you can access the full documentation for more examples from link below.
// http://hc.apache.org/httpcomponents-client-ga
// http://hc.apache.org/httpcomponents-client-ga/examples.html
public class SendSmsMain {
public static void main(String[] args) throws IOException {
SendSms();
}
public static void SendSms() throws IOException {
HttpClient httpclient = HttpClients.createDefault();
HttpPost httppost = new HttpPost("https://api.apitel.co/sms-batch");
String body = "{\"from\":\"ATSMS\"" +
", \"apiKey\":\"xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx\"" +
", \"apiSecret\":\"xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx\"" +
",\"messages\":[" +
"{\"to\":\"+661234567890\", \"text\":\"A first text message\"},"+
"{\"to\":\"+661234567891\", \"text\":\"A second text message\"}"+
"]}";
httppost.setEntity(new StringEntity(body, "UTF-8"));
httppost.addHeader("Content-Type", "application/json");
HttpResponse response = httpclient.execute(httppost);
HttpEntity entity = response.getEntity();
String responseBody = entity != null ? EntityUtils.toString(entity) : null;
int status = response.getStatusLine().getStatusCode();
if (status >= 200 && status < 300) {
System.out.println("Successful! Server responded with:" + responseBody);
} else {
System.out.println("Failed! Server responded with:" + responseBody);
}
}
}
using System;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
namespace apitel_test
{
class Program
{
static void Main(string[] args)
{
SendSmsAsync().Wait();
}
private static async Task SendSmsAsync()
{
using (HttpClient client = new HttpClient())
{
var url = "https://api.apitel.co/sms-batch";
var body = "{\"from\":\"ATSMS\"" +
", \"apiKey\":\"xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx\"" +
", \"apiSecret\":\"xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx\"" +
",\"messages\":[" +
"{\"to\":\"+661234567890\", \"text\":\"A first text message\"},"+
"{\"to\":\"+661234567891\", \"text\":\"A second text message\"}"+
"]}";
var payload = new StringContent(body, Encoding.UTF8, "application/json");
var response = await client.PostAsync(url, payload);
using (HttpContent content = response.Content)
{
string result = await content.ReadAsStringAsync();
if (response.StatusCode == System.Net.HttpStatusCode.OK)
{
Console.WriteLine("Successful! Server responded with:" + result);
}
else
{
Console.WriteLine("Failed! Server responded with:" + result);
}
}
}
}
}
}
Response
Response ถูกจัดรูปแบบเป็น JSON เมื่อมีการส่ง request สำเร็จ จะมีตอบกลับเป็น HTTP status code: 200 OK แต่ถ้าหากมีข้อผิดพลาดเกิดขึ้นก็จะมีตอบกลับเป็น HTTP status code: 400 Bad Request
Keys
ชื่อของชุดข้อความ
Examples
Response สำหรับส่งข้อความสำเร็จ
{
"id": 106,
"name": "batch#1",
"totalCount": 2,
"acceptedCount": 2,
"rejectedCount": 0
}
Response สำหรับส่งข้อความไม่สำเร็จ สิ่งนี้อาจเกิดขึ้นในกรณีระบุ content type ที่ไม่ถูกต้อง หรือ json syntax ไม่ถูกต้อง
{
"errors": {
"request": [
"Unable to parse request"
]
}
}
Response สำหรับส่งข้อความไม่สำเร็จ สิ่งนี้อาจเกิดขึ้นในกรณีไม่ระบุพารามิเตอร์ที่จำเป็น
{
"errors": {
"messages": "can't be empty."
}
}
Response สำหรับข้อความที่สามารถส่งสำเร็จบางส่วน โดยข้อความบางส่วนที่ส่งไม่สำเร็จจะมีข้อมูลบอกอันดับและข้อมูลว่าทำไมไม่สามารถส่งได่
{
"id": 11,
"name": "batch#1",
"totalCount": 2,
"acceptedCount": 1,
"rejectedCount": 1,
"errors": [{
"index": 1,
"text": "can't be empty"
}]
}
Batch SMS Status
สถานะของกลุ่มข้อความ (SMS Batch) จะถูกส่งผ่าน status webhook โดยแต่ละสถานะนั้นจะประกอบด้วยหมายเลขของ batch_id
SMS Batch Info
ในการแสดงข้อมูลของ Batch ให้ส่ง GET request ไปที่ /sms-batch/{id} endpoint
Request
URL | https://api.apitel.co/sms-batch/{id} |
Method | GET |
Parameters
Examples
curl -X GET 'https://api.apitel.co/sms-batch/127?apiKey=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx&apiSecret=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
const fetch = require('node-fetch');
const { URL } = require('url');
const url = new URL(`https://api.apitel.co/sms-batch/127`);
url.searchParams.append("apiKey", "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx");
url.searchParams.append("apiSecret", "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx");
fetch(url.href, {
method: 'GET',
headers: { 'Content-Type': 'application/json' }
})
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error(err));
$qs = '?apiKey=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
.'&apiSecret=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx';
$ch = curl_init('https://api.apitel.co/sms-batch/127'.$qs);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "GET");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$result = curl_exec($ch);
$http_code = curl_getinfo( $ch, CURLINFO_HTTP_CODE );
if ( $http_code == "200" ){
echo 'Successful! Server responded with:'.$result;
}else{
echo 'Failed! Server responded with:'.$result;
}
curl_close( $ch );
require 'net/http'
require 'uri'
require 'json'
url = "https://api.apitel.co/sms-batch/127"
qs = "?apiKey=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
qs += "&apiSecret=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
uri = URI.parse("#{url}#{qs}")
http = Net::HTTP.new(uri.host, uri.port)
request = Net::HTTP::Get.new(uri.request_uri)
response = http.request(request)
if response.is_a?(Net::HTTPSuccess)
puts "Successful! Server responded with: #{response.body }"
else
puts "Failed! Server responded with: #{response.body }"
end
import requests
params = {
'apiKey': "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
'apiSecret': "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
}
r = requests.get('https://api.apitel.co/sms-batch/127', params = params)
if r.status_code >= 200 and r.status_code < 300:
print('Successful! Server responded with:', r.json())
else:
print('Failed! Server responded with:', r.json())
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.utils.URIBuilder;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
// This example requires Apache HTTP Components dependency,
// you can access the full documentation for more examples from link below.
// http://hc.apache.org/httpcomponents-client-ga
// http://hc.apache.org/httpcomponents-client-ga/examples.html
public class ListSenderNameMain {
public static void main(String[] args) throws IOException, URISyntaxException {
ListSenderName();
}
public static void ListSenderName() throws IOException, URISyntaxException {
HttpClient httpclient = HttpClients.createDefault();
URI uri = new URIBuilder()
.setScheme("https")
.setHost("api.apitel.co")
.setPath("/sms-batch/127")
.setParameter("apiKey", "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx")
.setParameter("apiSecret", "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx")
.build();
HttpGet httpget = new HttpGet(uri);
HttpResponse response = httpclient.execute(httpget);
HttpEntity entity = response.getEntity();
String responseBody = entity != null ? EntityUtils.toString(entity) : null;
int status = response.getStatusLine().getStatusCode();
if (status >= 200 && status < 300) {
System.out.println("Successful! Server responded with:" + responseBody);
} else {
System.out.println("Failed! Server responded with:" + responseBody);
}
}
}
using System;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
namespace apitel_test
{
class Program
{
static void Main(string[] args)
{
ListSenderNameAsync().Wait();
}
private static async Task ListSenderNameAsync()
{
using (HttpClient client = new HttpClient())
{
var url = "https://api.apitel.co/sms-batch/127";
var qs = "?apiKey=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx";
qs += "&apiSecret=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx";
var response = await client.GetAsync(url + qs);
using (HttpContent content = response.Content)
{
string result = await content.ReadAsStringAsync();
if (response.StatusCode == System.Net.HttpStatusCode.OK)
{
Console.WriteLine("Successful! Server responded with:" + result);
}
else
{
Console.WriteLine("Failed! Server responded with:" + result);
}
}
}
}
}
}
Response
Response ถูกจัดรูปแบบเป็น JSON เมื่อมีการส่ง request สำเร็จ จะมีตอบกลับเป็น HTTP status code: 200 OK แต่ถ้าหากมีข้อผิดพลาดเกิดขึ้นก็จะมีตอบกลับเป็น HTTP status code: 400 Bad Request
Keys
Message object
Examples
Response สำหรับส่งข้อความสำเร็จ
{
"id": 127,
"name": "batch#2",
"totalCount": 3,
"acceptedCount": 3,
"rejectedCount": 0,
"sentCount": 0,
"deliveredCount": 0,
"undeliveredCount": 0,
"messages": [{
"id": 16987909,
"from": "ATSMS",
"to": "+661234567890",
"state": "DELIVERED"
},
{
"id": 16987910,
"from": "ATSMS",
"to": "+661234567891",
"state": "DELIVERED"
},
{
"id": 16987911,
"from": "ATSMS",
"to": "+661234567892",
"state": "DELIVERED"
}
],
"isActive": true
}
ชื่อผู้ส่ง
API สำหรับการส่งคำขอเพื่อเพิ่มและลบชื่อผู้ส่ง (Sender name) รวมถึงการแสดงรายชื่อผู้ส่งของคุณ
การส่งคำขอชื่อผู้ส่ง
ในการส่งคำขอเพื่อเพิ่มชื่อผู้ส่งให้ส่งคำขอ POSTไปที่ /sender_names endpoint
การส่งคำขอ
URL | https://api.apitel.co/sender_names |
Method | POST |
Content type | application/x-www-form-urlencoded หรือ application/json |
พารามิเตอร์
ตัวอย่าง
curl -i -X POST https://api.apitel.co/sender_names \
-H "Content-Type:application/json" \
-d \
'{
"name": "goodco",
"purpose": "NOTIFICATION",
"exampleMsg": "Your OTP is 123456 (Ref. ABCD) 18:00",
"ownerType": "WEBSITE",
"owner": "http://apitel.co",
"apiKey": "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
"apiSecret": "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
}'
const fetch = require('node-fetch');
const body = {
name: "goodco",
purpose: "NOTIFICATION",
exampleMsg: "Your OTP is 123456 (Ref. ABCD) 18:00",
ownerType: "WEBSITE",
owner: "http://apitel.co",
apiKey: "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
apiSecret: "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
};
fetch('https://api.apitel.co/sender_names', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(body)
})
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error(err));
$data = array(
"name" => "goodco",
"purpose" => "NOTIFICATION",
"exampleMsg" => "Your OTP is 123456 (Ref. ABCD) 18:00",
"ownerType" => "WEBSITE",
"owner" => "http://apitel.co",
"apiKey" => "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
"apiSecret" => "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
);
$data_string = json_encode($data);
$ch = curl_init('https://api.apitel.co/sender_names');
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "POST");
curl_setopt($ch, CURLOPT_POSTFIELDS, $data_string);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json',
'Content-Length: ' . strlen($data_string))
);
$result = curl_exec($ch);
$http_code = curl_getinfo( $ch, CURLINFO_HTTP_CODE );
if ( $http_code == "200" ){
echo 'Successful! Server responded with:'.$result;
}else{
echo 'Failed! Server responded with:'.$result;
}
curl_close( $ch );
require 'net/http'
require 'uri'
require 'json'
uri = URI.parse("https://api.apitel.co/sender_names")
header = {'Content-Type': 'application/json'}
body = {
name: "goodco",
purpose: "NOTIFICATION",
exampleMsg: "Your OTP is 123456 (Ref. ABCD) 18:00",
ownerType: "WEBSITE",
owner: "http://apitel.co",
apiKey: "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
apiSecret: "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
}
http = Net::HTTP.new(uri.host, uri.port)
request = Net::HTTP::Post.new(uri.request_uri, header)
request.body = body.to_json
response = http.request(request)
if response.is_a?(Net::HTTPSuccess)
puts "Successful! Server responded with: #{response.body }"
else
puts "Failed! Server responded with: #{response.body }"
end
import requests
body = {
'name': "goodco",
'purpose': "NOTIFICATION",
'exampleMsg': "Your OTP is 123456 (Ref. ABCD) 18:00",
'ownerType': "WEBSITE",
'owner': "http://apitel.co",
'apiKey': "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
'apiSecret': "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
}
r = requests.post('https://api.apitel.co/sender_names', json=body)
if r.status_code >= 200 and r.status_code < 300:
print('Successful! Server responded with:', r.json())
else:
print('Failed! Server responded with:', r.json())
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.io.IOException;
// This example requires Apache HTTP Components dependency,
// you can access the full documentation for more examples from link below.
// http://hc.apache.org/httpcomponents-client-ga
// http://hc.apache.org/httpcomponents-client-ga/examples.html
public class RequestSenderNameMain {
public static void main(String[] args) throws IOException {
RequestSenderName();
}
public static void RequestSenderName() throws IOException {
HttpClient httpclient = HttpClients.createDefault();
HttpPost httppost = new HttpPost("https://api.apitel.co/sender_names");
String body = "{\"name\":\"goodco\"" +
",\"purpose\":\"NOTIFICATION\"" +
",\"exampleMsg\":\"Your OTP is 123456 (Ref. ABCD) 18:00\"" +
",\"ownerType\":\"WEBSITE\"" +
",\"owner\":\"http://apitel.co\"" +
",\"apiKey\":\"xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"\"" +
",\"apiSecret\":\"xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"\"}";
httppost.setEntity(new StringEntity(body, "UTF-8"));
httppost.addHeader("Content-Type", "application/json");
HttpResponse response = httpclient.execute(httppost);
HttpEntity entity = response.getEntity();
String responseBody = entity != null ? EntityUtils.toString(entity) : null;
int status = response.getStatusLine().getStatusCode();
if (status >= 200 && status < 300) {
System.out.println("Successful! Server responded with:" + responseBody);
} else {
System.out.println("Failed! Server responded with:" + responseBody);
}
}
}
using System;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
namespace apitel_test
{
class Program
{
static void Main(string[] args)
{
RequestSenderNameAsync().Wait();
}
private static async Task RequestSenderNameAsync()
{
using (HttpClient client = new HttpClient())
{
var url = "https://api.apitel.co/sender_names";
var body = "{\"name\":\"goodco\"" +
",\"purpose\":\"NOTIFICATION\"" +
",\"exampleMsg\":\"Your OTP is 123456 (Ref. ABCD) 18:00\"" +
",\"ownerType\":\"WEBSITE\"" +
",\"owner\":\"http://apitel.co\"" +
",\"apiKey\":\"xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx\"" +
",\"apiSecret\":\"xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx\"}";
var payload = new StringContent(body, Encoding.UTF8, "application/json");
var response = await client.PostAsync(url, payload);
using (HttpContent content = response.Content)
{
string result = await content.ReadAsStringAsync();
if (response.StatusCode == System.Net.HttpStatusCode.OK)
{
Console.WriteLine("Successful! Server responded with:" + result);
}
else
{
Console.WriteLine("Failed! Server responded with:" + result);
}
}
}
}
}
}
การตอบรับ
การตอบรับจะถูกจัดรูปแบบเป็น JSON เมื่อมีการส่งการส่งคำขอสำเร็จ จะมีตอบกลับเป็น HTTP status code: 200 OK แต่ถ้าหากมีข้อผิดพลาดเกิดขึ้นก็จะมีตอบกลับเป็น HTTP status code: 400 Bad Request
รหัส
ตัวอย่าง
การตอบรับเมื่อการส่งคำขอสำเร็จ
{
"name": "goodco",
"pending": true,
"reliable": false,
"default": false
}
รายชื่อผู้ส่ง
ในการแสดงรายชื่อผู้ส่งให้ส่งคำขอ GETไปที่ /sender_names endpoint
การร้องขอ
URL | https://api.apitel.co/sender_names |
Method | GET |
พารามิเตอร์
ตัวอย่าง
curl -X GET 'https://api.apitel.co/sender_names?apiKey=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx&apiSecret=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
const fetch = require('node-fetch');
const { URL } = require('url');
const url = new URL(`https://api.apitel.co/sender_names`);
url.searchParams.append("apiKey", "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx");
url.searchParams.append("apiSecret", "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx");
fetch(url.href, {
method: 'GET',
headers: { 'Content-Type': 'application/json' }
})
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error(err));
$qs = '?apiKey=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
.'&apiSecret=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx';
$ch = curl_init('https://api.apitel.co/sender_names'.$qs);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "GET");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$result = curl_exec($ch);
$http_code = curl_getinfo( $ch, CURLINFO_HTTP_CODE );
if ( $http_code == "200" ){
echo 'Successful! Server responded with:'.$result;
}else{
echo 'Failed! Server responded with:'.$result;
}
curl_close( $ch );
require 'net/http'
require 'uri'
require 'json'
url = "https://api.apitel.co/sender_names"
qs = "?apiKey=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
qs += "&apiSecret=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
uri = URI.parse("#{url}#{qs}")
http = Net::HTTP.new(uri.host, uri.port)
request = Net::HTTP::Get.new(uri.request_uri)
response = http.request(request)
if response.is_a?(Net::HTTPSuccess)
puts "Successful! Server responded with: #{response.body }"
else
puts "Failed! Server responded with: #{response.body }"
end
import requests
params = {
'apiKey': "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
'apiSecret': "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
}
r = requests.get('https://api.apitel.co/sender_names', params = params)
if r.status_code >= 200 and r.status_code < 300:
print('Successful! Server responded with:', r.json())
else:
print('Failed! Server responded with:', r.json())
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.utils.URIBuilder;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
// This example requires Apache HTTP Components dependency,
// you can access the full documentation for more examples from link below.
// http://hc.apache.org/httpcomponents-client-ga
// http://hc.apache.org/httpcomponents-client-ga/examples.html
public class ListSenderNameMain {
public static void main(String[] args) throws IOException, URISyntaxException {
ListSenderName();
}
public static void ListSenderName() throws IOException, URISyntaxException {
HttpClient httpclient = HttpClients.createDefault();
URI uri = new URIBuilder()
.setScheme("https")
.setHost("api.apitel.co")
.setPath("/sender_names")
.setParameter("apiKey", "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx")
.setParameter("apiSecret", "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx")
.build();
HttpGet httpget = new HttpGet(uri);
HttpResponse response = httpclient.execute(httpget);
HttpEntity entity = response.getEntity();
String responseBody = entity != null ? EntityUtils.toString(entity) : null;
int status = response.getStatusLine().getStatusCode();
if (status >= 200 && status < 300) {
System.out.println("Successful! Server responded with:" + responseBody);
} else {
System.out.println("Failed! Server responded with:" + responseBody);
}
}
}
using System;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
namespace apitel_test
{
class Program
{
static void Main(string[] args)
{
ListSenderNameAsync().Wait();
}
private static async Task ListSenderNameAsync()
{
using (HttpClient client = new HttpClient())
{
var url = "https://api.apitel.co/sender_names";
var qs = "?apiKey=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx";
qs += "&apiSecret=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx";
var response = await client.GetAsync(url + qs);
using (HttpContent content = response.Content)
{
string result = await content.ReadAsStringAsync();
if (response.StatusCode == System.Net.HttpStatusCode.OK)
{
Console.WriteLine("Successful! Server responded with:" + result);
}
else
{
Console.WriteLine("Failed! Server responded with:" + result);
}
}
}
}
}
}
การตอบรับ
การตอบรับจะถูกจัดรูปแบบเป็น JSON รายชื่อผู้ส่งจะเป็นชุดข้อมูล Array ที่ประกอบไปด้วยรายละเอียดของชื่อผู้ส่งโดยมีรหัสดังต่อไปนี้
รหัส
ตัวอย่าง
การตอบรับเมื่อแสดงรายชื่อผู้ส่งสำเร็จ
{
"sender_names": [
{
"name": "goodco",
"pending": true,
"reliable": false,
"default": false
},
{
"name": "verygoodco",
"pending": false,
"reliable": true,
"default": true
}
]
}
ลบชื่อผู้ส่ง
ในการลบชื่อผู้ส่งให้ส่งคำขอ DELTEไปที่ /sender_names endpoint
การส่งคำขอ
URL | https://api.apitel.co/sender_names/{name} |
Method | DELETE |
พารามิเตอร์
ตัวอย่าง
curl -X DELETE 'https://api.apitel.co/sender_names/goodco?apiKey=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx&apiSecret=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
const fetch = require('node-fetch');
const { URL } = require('url');
const sender_name = 'goodco';
const url = new URL(`https://api.apitel.co/sender_names/${sender_name}`);
url.searchParams.append("apiKey", "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx");
url.searchParams.append("apiSecret", "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx");
fetch(url.href, {
method: 'DELETE',
headers: { 'Content-Type': 'application/json' }
})
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error(err));
$sender_name = 'goodco';
$qs = '?apiKey=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
.'&apiSecret=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx';
$ch = curl_init('https://api.apitel.co/sender_names/'.$sender_name.$qs);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "DELETE");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$result = curl_exec($ch);
$http_code = curl_getinfo( $ch, CURLINFO_HTTP_CODE );
if ( $http_code == "200" ){
echo 'Successful! Server responded with:'.$result;
}else{
echo 'Failed! Server responded with:'.$result;
}
curl_close( $ch );
require 'net/http'
require 'uri'
url = "https://api.apitel.co/sender_names"
sender_name = "goodco"
qs = "?apiKey=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
qs += "&apiSecret=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
uri = URI.parse("#{url}/#{sender_name}#{qs}")
http = Net::HTTP.new(uri.host, uri.port)
request = Net::HTTP::Delete.new(uri.request_uri)
response = http.request(request)
if response.is_a?(Net::HTTPSuccess)
puts "Successful! Server responded with: #{response.body }"
else
puts "Failed! Server responded with: #{response.body }"
end
import requests
params = {
'apiKey': "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
'apiSecret': "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
}
r = requests.delete('https://api.apitel.co/sender_names/goodco', params = params)
if r.status_code >= 200 and r.status_code < 300:
print('Successful! Server responded with:', r.json())
else:
print('Failed! Server responded with:', r.json())
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpDelete;
import org.apache.http.client.utils.URIBuilder;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
// This example requires Apache HTTP Components dependency,
// you can access the full documentation for more examples from link below.
// http://hc.apache.org/httpcomponents-client-ga
// http://hc.apache.org/httpcomponents-client-ga/examples.html
public class DelSenderNameMain {
public static void main(String[] args) throws IOException, URISyntaxException {
DelSenderName();
}
public static void DelSenderName() throws IOException, URISyntaxException {
HttpClient httpclient = HttpClients.createDefault();
URI uri = new URIBuilder()
.setScheme("https")
.setHost("api.apitel.co")
.setPath("/sender_names/goodco")
.setParameter("apiKey", "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx")
.setParameter("apiSecret", "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx")
.build();
HttpDelete httpdelete = new HttpDelete(uri);
HttpResponse response = httpclient.execute(httpdelete);
HttpEntity entity = response.getEntity();
String responseBody = entity != null ? EntityUtils.toString(entity) : null;
int status = response.getStatusLine().getStatusCode();
if (status >= 200 && status < 300) {
System.out.println("Successful! Server responded with:" + responseBody);
} else {
System.out.println("Failed! Server responded with:" + responseBody);
}
}
}
using System;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
namespace apitel_test
{
class Program
{
static void Main(string[] args)
{
DelSenderNameAsync().Wait();
}
private static async Task DelSenderNameAsync()
{
using (HttpClient client = new HttpClient())
{
var url = "https://api.apitel.co/sender_names/goodco";
var qs = "?apiKey=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx";
qs += "&apiSecret=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx";
var response = await client.DeleteAsync(url + qs);
using (HttpContent content = response.Content)
{
string result = await content.ReadAsStringAsync();
if (response.StatusCode == System.Net.HttpStatusCode.OK)
{
Console.WriteLine("Successful! Server responded with:" + result);
}
else
{
Console.WriteLine("Failed! Server responded with:" + result);
}
}
}
}
}
}
การตอบรับ
การตอบรับจะถูกจัดรูปแบบเป็น JSON เป็นข้อมูลรายละเอียดของซื้อผู้ส่งที่ถูกลบ
รหัส
ตัวอย่าง
การตอบรับเมื่อมีการลบชื่อผู้ส่งสำเร็จ
{
"name": "goodco",
"pending": false,
"reliable": false,
"default": false
}